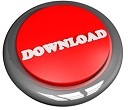
getLast - Returns the data of the last element.getFirst - Returns the data of the first element.removeFirst - Removes the first element from Linked List.size - Returns the number of elements in the linked list.display - Prints the elements of a linked list from front to end in a single line.addLast - adds a new element with given value to the end of Linked List.You are given a partially written LinkedList class.Reverse Linked List (pointer - Recursive) While inserting it at the end, the second last node of the list should point to the new node and the new node will point to NULL.Remove Duplicates In A Sorted Linked Listĭisplay Reverse (recursive) - Linked List Similar steps should be taken if the node is being inserted at the beginning of the list. We can keep that in memory otherwise we can simply deallocate memory and wipe off the target node completely. Now, using the following code, we will remove what the target node is pointing at. This will remove the link that was pointing to the target node. The left (previous) node of the target node now should point to the next node of the target node − First, locate the target node to be removed, by using searching algorithms. We shall learn with pictorial representation. Struct node *lk = (struct node*) malloc(sizeof(struct node)) ĭeletion is also a more than one step process. Assign the head to the newly added node.įollowing are the implementations of this operation in various programming languages − If the list is empty, add the data to the node and assign the head pointer to it.ĥ If the list is not empty, add the data to a node and link to the current head. In this operation, we are adding an element at the beginning of the list. They are explained as follows − Insertion at Beginning Insertion in linked list can be done in three different ways. This will put the new node in the middle of the two. Now, the next node at the left should point to the new node. Imagine that we are inserting a node B (NewNode), between A (LeftNode) and C (RightNode). First, create a node using the same structure and find the location where it has to be inserted. Search − Searches an element using the given key.ĭelete − Deletes an element using the given key.Īdding a new node in linked list is a more than one step activity. Insertion − Adds an element at the beginning of the list.ĭeletion − Deletes an element at the beginning of the list. These operations are performed on Singly Linked Lists as given below − The basic operations in the linked lists are insertion, deletion, searching, display, and deleting an element at a given key. Since the last node and the first node of the circular linked list are connected, the traversal in this linked list will go on forever until it is broken. Circular Linked ListsĬircular linked lists can exist in both singly linked list and doubly linked list. The list is traversed twice as the nodes in the list are connected to each other from both sides. Doubly Linked Listsĭoubly Linked Lists contain three “buckets” in one node one bucket holds the data and the other buckets hold the addresses of the previous and next nodes in the list. Traversals can be done in one direction only as there is only a single link between two nodes of the same list. Singly linked lists contain two “buckets” in one node one bucket holds the data and the other bucket holds the address of the next node of the list. Last link carries a link as null to mark the end of the list.įollowing are the various types of linked list. Linked List contains a link element called first (head).Įach link carries a data field(s) and a link field called next.Įach link is linked with its next link using its next link. Linked list can be visualized as a chain of nodes, where every node points to the next node.Īs per the above illustration, following are the important points to be considered. It can either be singly linked or doubly linked. Singly Linked List − The nodes only point to the address of the next node in the list.ĭoubly Linked List − The nodes point to the addresses of both previous and next nodes.Ĭircular Linked List − The last node in the list will point to the first node in the list. Any amount of data can be stored in it and can be deleted from it.
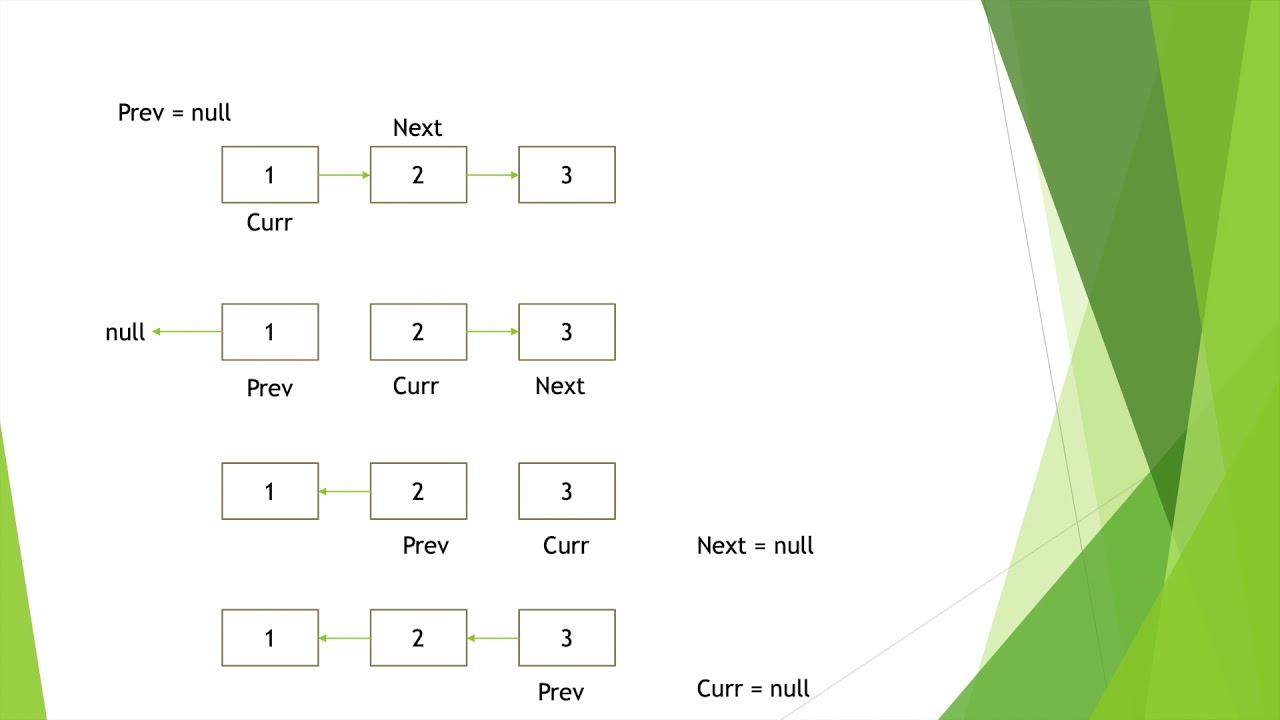
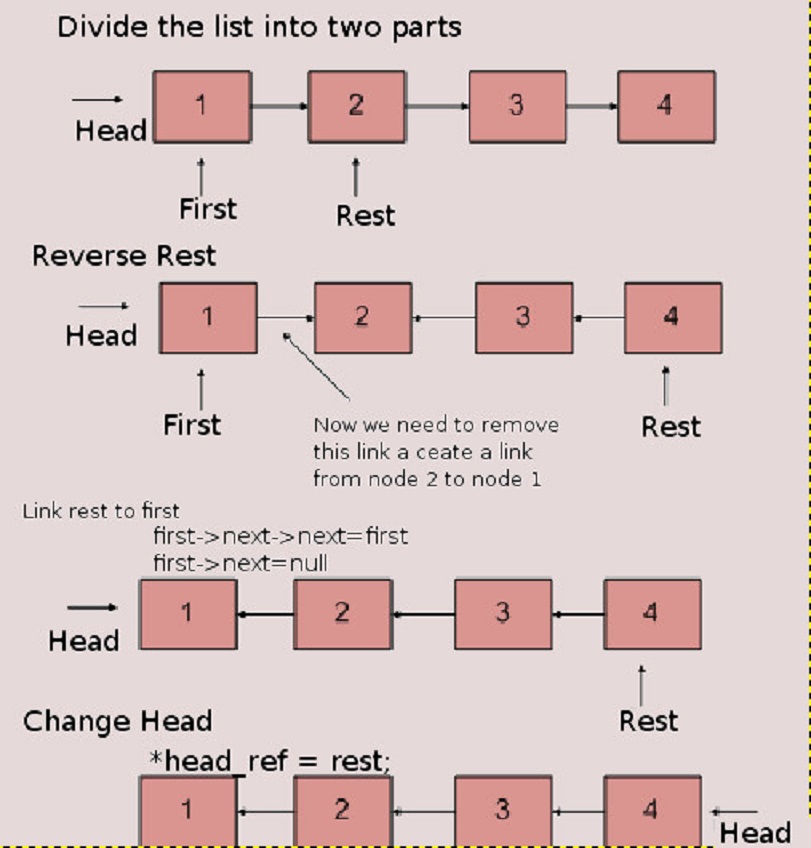
In the case of arrays, the size is limited to the definition, but in linked lists, there is no defined size. These nodes consist of the data to be stored and a pointer to the address of the next node within the linked list. If arrays accommodate similar types of data types, linked lists consist of elements with different data types that are also arranged sequentially.Ī linked list is a collection of “nodes” connected together via links.
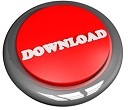